Fundamental Programme -C, C++, Data Structure
Fundamental Programme -C, C++, Data Structure
C And C++ Data structures in the context of programming languages like C and C++ refer to the way data is organized and stored in computer memory. They provide a means of efficiently managing and manipulating data for various computational tasks.
Here are definitions of data structures in C and C++:
Data Structure in C: A data structure in C is a specific format for organizing and storing data efficiently in memory. It defines how data elements are stored, accessed, and manipulated. Data structures in C include arrays, structures, linked lists, stacks, queues, trees, and graphs, among others. These data structures enable programmers to perform various operations on data, such as insertion, deletion, searching, and sorting, based on the structure’s design and the problem being solved.
Data Structure in C++: Data structures in C++ encompass the same concepts as in C but often benefit from the features of the C++ programming language. In C++, data structures can be implemented using classes and objects. Additionally, C++ includes the Standard Template Library (STL), which provides a collection of pre-implemented data structures like vectors, lists, stacks, queues, maps, sets, and more. These data structures in C++ are often templates, allowing for generic programming and providing reusable solutions for a wide range of data organization and manipulation tasks.
In summary, data structures in C and C++ refer to the techniques and formats used to manage data efficiently. While C relies on custom implementations, C++ offers the advantage of using classes and leverages the STL to provide a standardized and versatile set of data structures that simplify the process of working with data.
How are data structures used?
Programmers could define their own data structures using early programming languages like Fortran, C, and C++. A large number of programming languages nowadays come with a large number of built-in data structures for organizing code and data. Common coding structures for storing and retrieving data include lists and dictionaries in Python and arrays and objects in JavaScript.
Some examples of how data structures are used include the following:
Data exchange: The structure of information shared by applications, like TCP/IP packets, is defined by data structures.

Ordering and sorting: Character strings used as tags can be sorted efficiently using data structures like binary search trees, also called an ordered or sorted binary tree. Programmers can handle items arranged based on a specified priority by using data structures like priority queues.

Searching: Hash tables, B-trees, and binary search trees are some of the index creation techniques that can speed up the process of locating a particular item.
Indexing: To index objects, like those kept in a database, even more complex data structures like B-trees are utilized.
Data types: There are 2 types of Data structure that is:
1.Primitive Data Structure 2. Non Primitive Data Structure
1.Primitive Data Structure: The primitive, or base, data types are the building blocks of data structures, if data structures are the building blocks of algorithms and computer programs.
The following are examples of common base data types:
Boolean: Logical values that can be true or false are stored there.
Integer: which keeps track of a range of counting numbers, or mathematical integers. The range of values stored in different sized integers varies. For example, an unsigned long 32-bit integer can store values from 0 to 4,294,967,295, while a signed 8-bit integer can store values from -128 to 127.
Floating-point numbers: Real numbers are represented formulaically by floating-pointing numbers.
Fixed-point numbers: Certain programming languages employ fixed-point numbers, which manage real values as digits to the left and right of the decimal point.
Character: is a mapping of integer values to symbols that makes use of symbols.
Pointers: Reference values that point to other values are called pointers.
String: Strings are either managed by an integer length field or are an array of characters followed by a stop code, typically a “0” value.
2.Non Primitive Data Structure: Non-primitive data structures are data structures that are not directly built into the programming language but are instead implemented using primitive data types and other data structures. These include arrays, linked lists, stacks, queues, trees, graphs, and more. They are used to store and manage collections of data in a more complex and specialized manner compared to primitive data types like integers, floats, and characters.
Non primitive data structure are also have two types:
1. Linear Data Structure 2. Non Linear Data Structure
Linear Data Structure: In non-primitive data structures, a linear data structure refers to a data organization where data elements are arranged in a linear order, following a sequence. This means each element has a unique predecessor and successor, with the exception of the first and last elements. Common examples include arrays, lists, stacks, and queues.
Non Linear Data Structures: In the context of non-primitive data structures, a non-linear data structure refers to a data organization where data elements are not arranged sequentially. This means that each element may be connected to one or more other elements in a way that does not form a sequence. Examples of non-linear data structures include trees and graphs.
The data structure type used in a particular situation is determined by the type of operations that will be required or the kinds of algorithms that will be applied. The various data structure types include the following:
Array: Arrays can have fixed or flexible lengths, and they store a group of items at nearby memory locations that are all of the same type. This provides an index to easily calculate or retrieve the position of any element in the array.
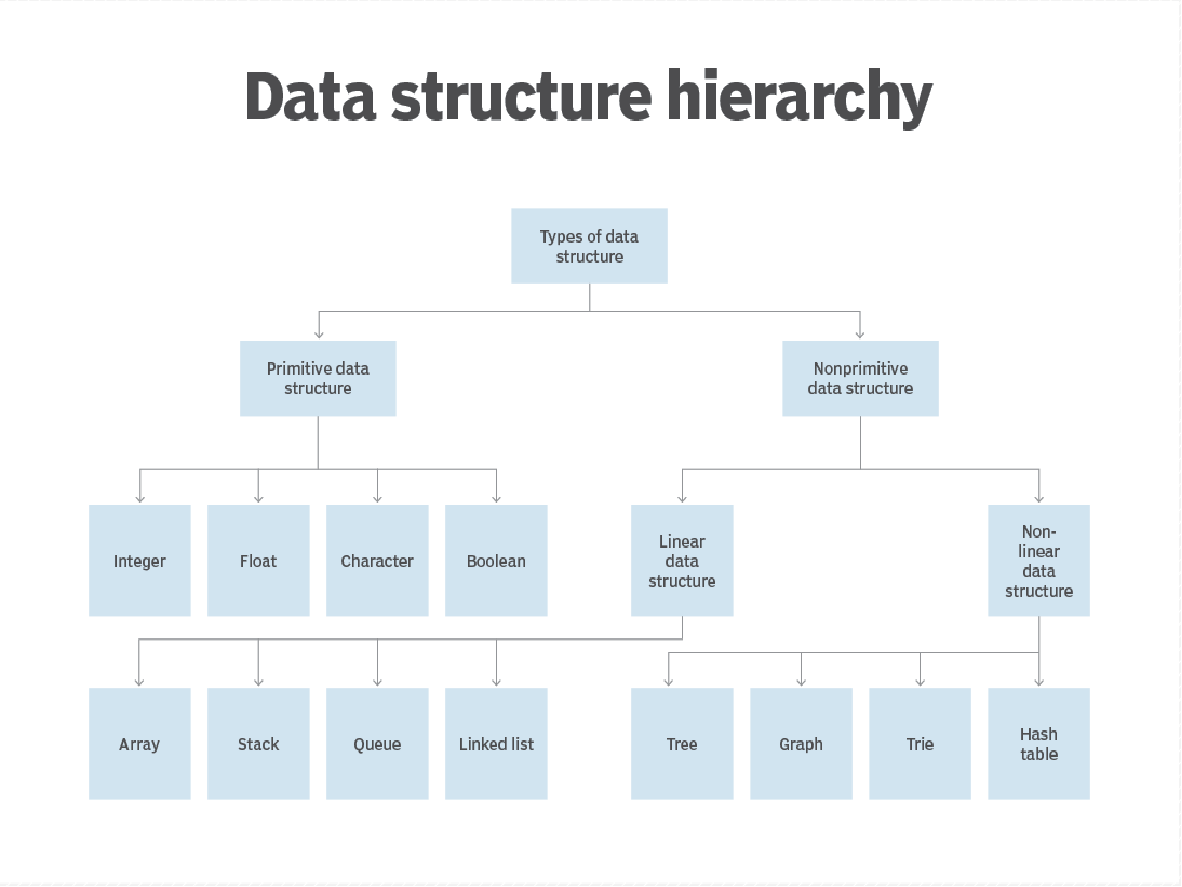
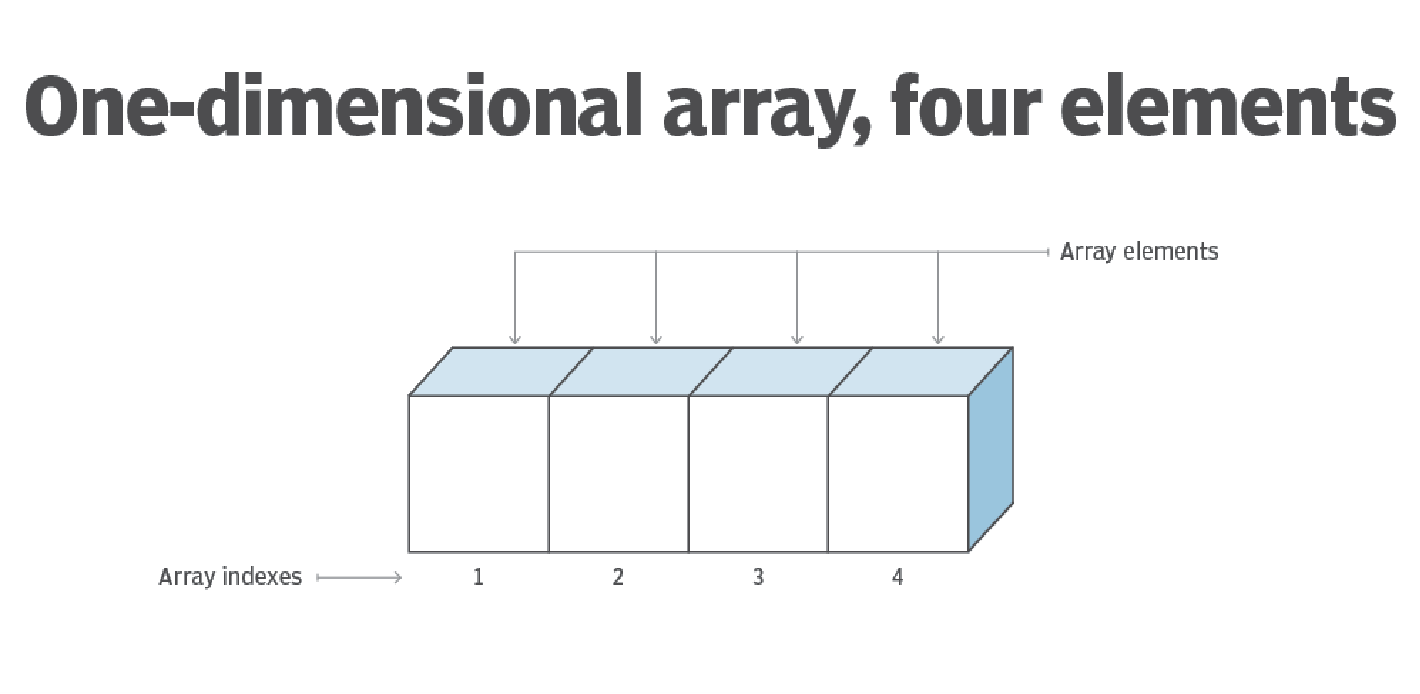
An array can hold a collection of integers, floating-point numbers, strings or even other arrays.
Stack: A stack stores a collection of items in the linear order that operations are applied. This order could be last in, first out (LIFO) or first in, first out (FIFO).
Queue: A queue stores a collection of items like a stack; however, the operation order can only be first in, first out.
Linked list: An array of items is kept in a linear order in a linked list. A linked list’s elements, or nodes, are composed of a data item and a reference, or link, to the list’s subsequent item.
Linked list data structures are a set of nodes that contain data and the address or a pointer to the next node.
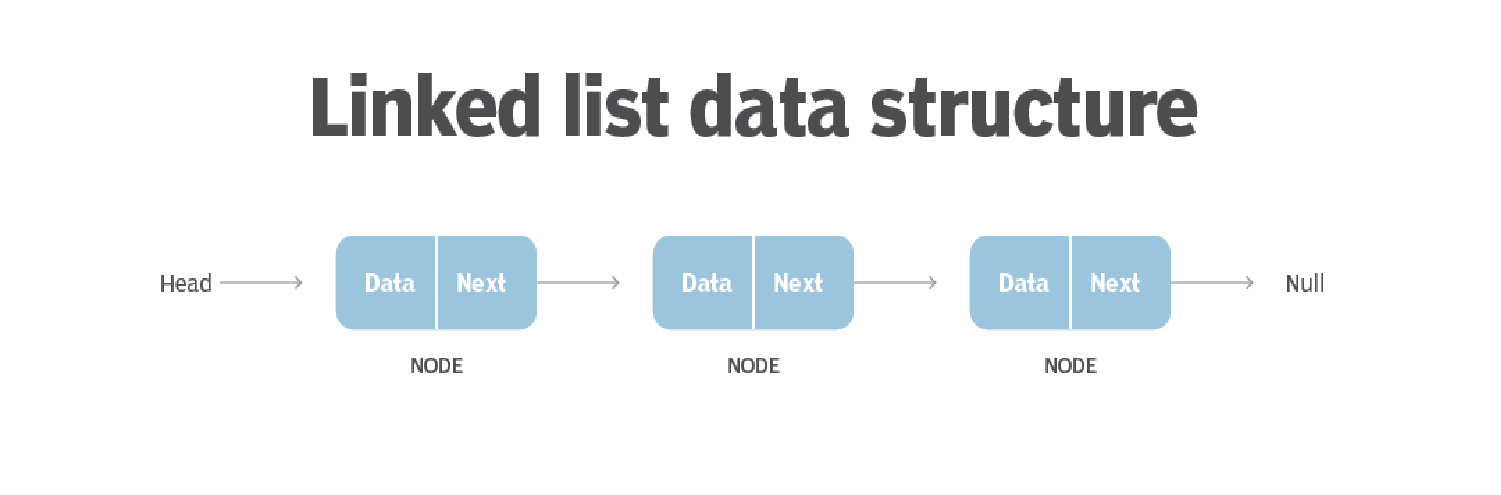
Tree: An abstract, hierarchical collection of items is stored in a tree. Every node has a key value assigned to it, and parent nodes are connected to child nodes, or sub nodes. All of the nodes in the tree have a single root node as their ancestor.
Heap: A heap is a tree-based structure where the associated key value of each parent node is greater than or equal to the key values of any of its offspring.
Chart : A graph uses nonlinear storage to hold a group of items. A finite set of nodes, also referred to as vertices, and the lines that connect them, also referred to as edges, comprise a graph. These are helpful for simulating actual systems, like computer networks.
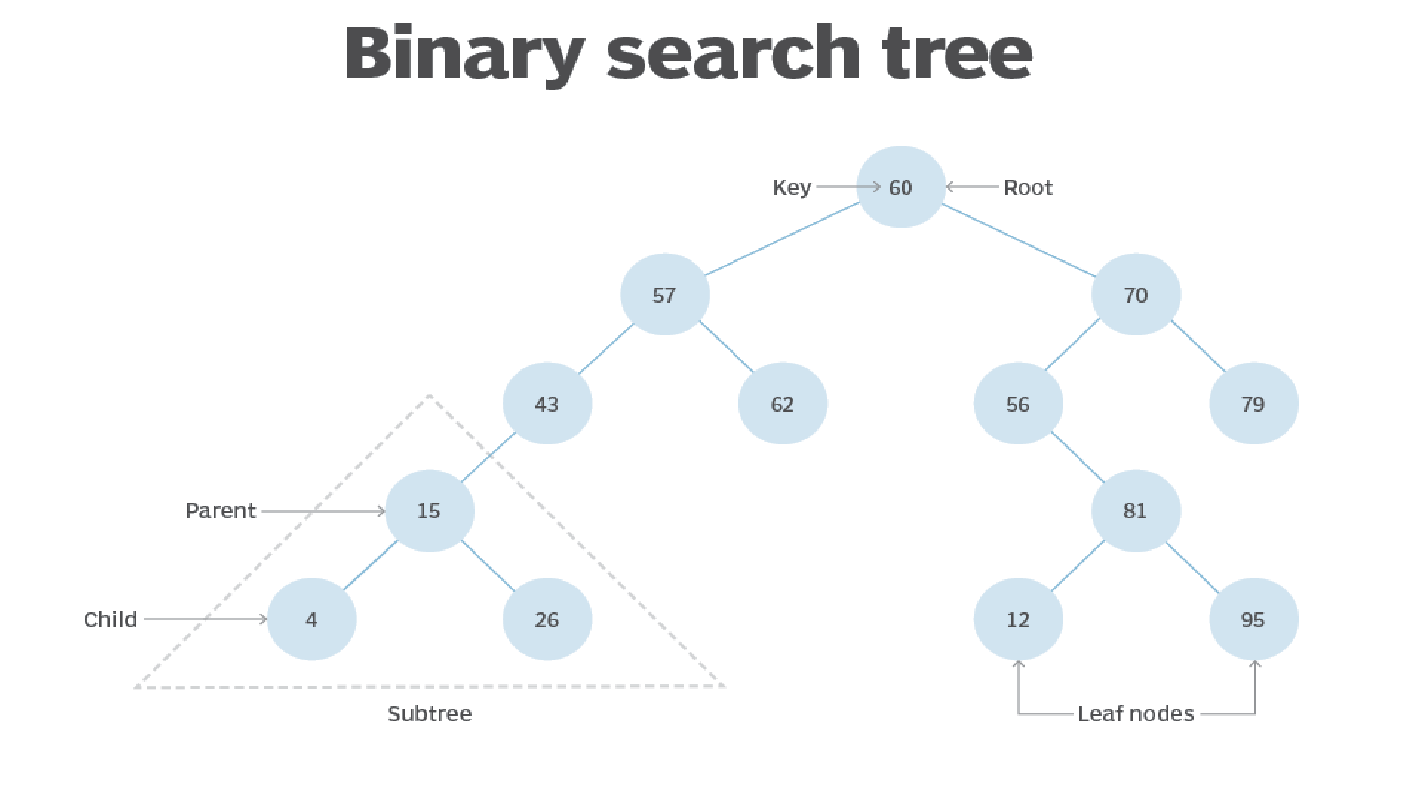
Trie: A trie, sometimes called a keyword tree, is a type of data structure used to store strings as individual data items that can be arranged visually in a graph.
Hash table: A hash table, sometimes referred to as a hash map, is a collection of items plotted as keys to values in an associative array. A hash table transforms an index into an array of buckets containing the desired data item using a hash function.
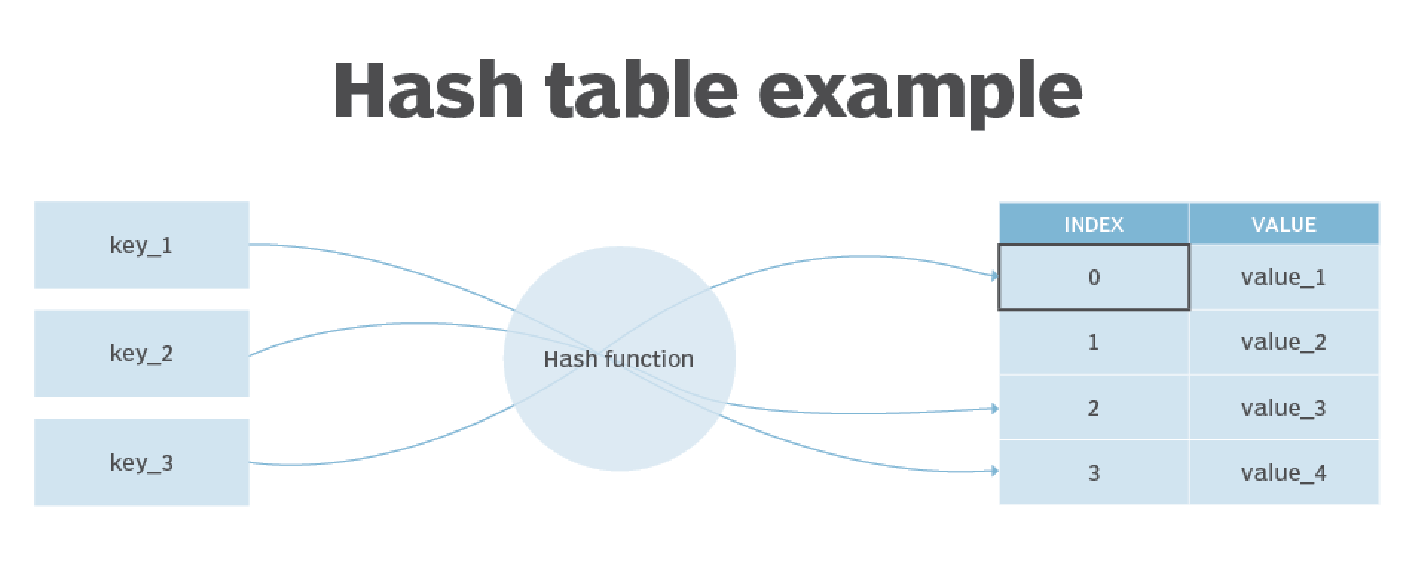
Why are data structures important?
However, in order to make processing easier, applications that produce, alter, and absorb data must comprehend how data should be arranged. Data structures enable the efficient use, persistence, and sharing of data by logically assembling the data elements. They offer a formal model that explains the arrangement of the data elements.
Applications with more complexity are built using data structures as their building blocks. They are created by assembling data components into a logical unit that pertains to the algorithm or application and represents an abstract data type. A “customer name” made up of the character strings for “first name,” “middle name,” and “last name” is an illustration of an abstract data type.
Selecting the appropriate data structure for each task is just as important as using data structures. Inappropriate data structure selection may lead to sluggish programs or unresponsive code. These five considerations are suggested by SCODEEN Global, while choosing a data structure:
1. What kind of data is going to be stored?
2. What goal will that information perform?
3. Where should data be stored, after it is created?
4. Which method of data organization works best?
5. Which memory and storage reservation management variables need to be taken into account?
Job Roles and Opportunities:
Data scientist
Business analyst
Database administrator
Analytics manager
Machine Learning Engineer
Senior Software Engineer
Investment Banker
Chief Executive Officer (CEO)
Engineering Manager
IT Manager
Financial Analyst
Information Systems Security Manager
DevOps Engineer
Enterprise Architect
Software Developer
Telecommunications Engineer
Performance Optimization Specialist
Firmware Engineer
Expected Package in Data Structure:
An employee with knowledge of data structures makes, on average, ₹20.6 lakhs. Data Structure candidates from SCODEEN Global have received packages ranging from ₹13 Lakhs to 35 lakhs per Annum.
Important Skills of C and C ++ Data Structure:
Proficient In Typing
Understanding Of Pointers and references
Memory Management
Data Structure Implementation
Algorithm Thinking
Problem Solving
Debugging and Testing Skills
Efficient Code Design
Optimization Techniques
Understanding Of Data Structure Complexity
Object-Oriented Programming
Error Handling
Compiler Specifies
Knowledge Of Basic Software
Communication Skills- Verbal And Written
Accuracy And Attention To Detail
Operating Knowledge Of Standard Office Equipment
Organizational and Time Management Skills
Basic Research and Collection Of Data Skills
Basic Understanding Of Database Structure
Teamwork
Characteristics of Data structures:
Linear or non-linear: If the data items are organized in an unordered sequence, like with a graph, or in sequential order, like with an array, this characteristic describes it.
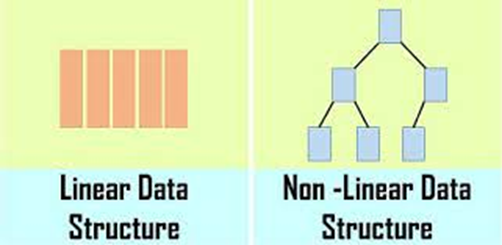
Homogeneous or Heterogeneous: This feature indicates whether or not every data item in a certain repository belongs to the same type. A collection of elements in an array or of different types, like a class specification in Java or an abstract data type defined as a structure in C, are two examples
Static or dynamic: This feature explains the compilation process for the data structures. At compile time, the sizes, structures, and memory locations of static data structures are fixed. Depending on their intended use, dynamic data structures can have varying sizes, structures, and memory locations.
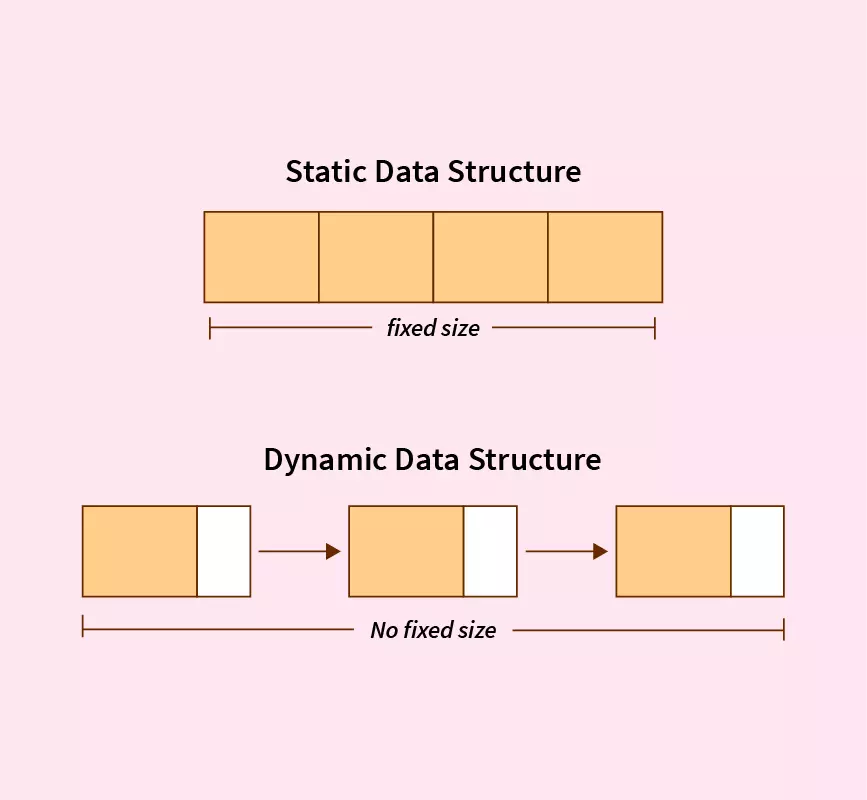
Course Highlights/ Details:
Learn Data Structure & Get Placement Opportunities.
Suited for Fresher’s & Recent Graduates.
Live Online Classes by Highly Experienced Faculties.
4-Month Full-Time Program.
Certificate Of Completion.
Decision Oriented Program of Analysis.
Hands on Experience with Real- Life Case Studies.
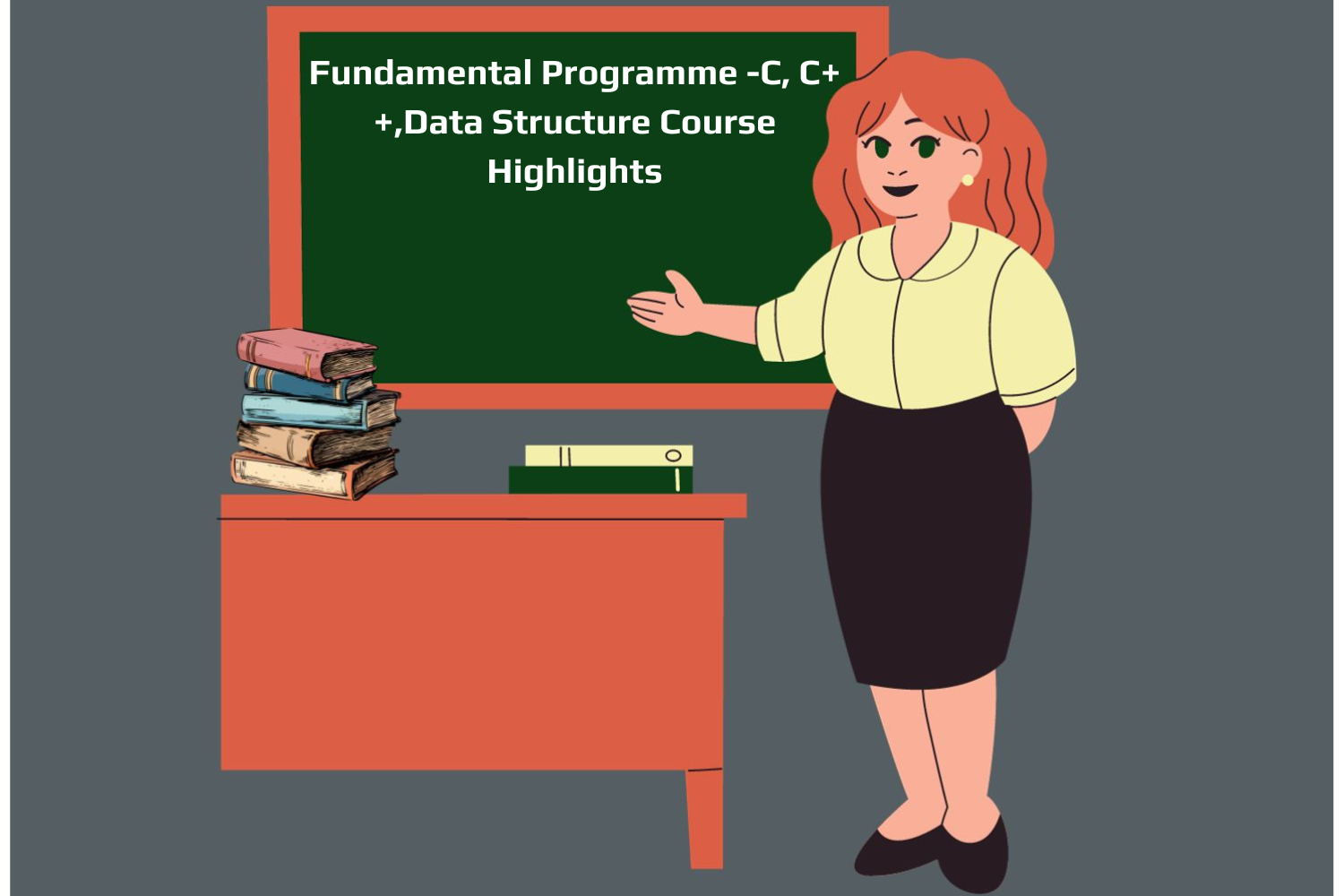
Conclusion:
This course covered the basics of data structures. With this we have only scratched the surface. Although we have built a good foundation to move ahead.
In conclusion, proficiency in C and C++ for data structures is highly beneficial and sought after in the field of software development and computer programming. These languages offer the flexibility and control required for implementing complex data structures efficiently. Understanding the nuances of memory management, pointers, and algorithms in C and C++ is crucial for developing robust and optimized data structure implementations. Moreover, the ability to analyze and optimize the performance of data structure operations is key to creating high-performance software applications. Overall, a strong grasp of C and C++ in the context of data structures is invaluable for those aiming to build efficient and reliable software solutions.