UNIT TESTING
Unit testing is a critical software development practice that involves the systematic examination of individual components, or “units,” of a computer program. These units are typically the smallest testable parts of the code, such as functions, methods, or classes. The primary objective of unit testing is to verify that each unit operates correctly in isolation, free from the influence of external dependencies. Unit tests are automated and can be executed without manual intervention, ensuring consistency and repeatability. They are designed to be fast, enabling frequent testing and early bug detection. By isolating specific code segments and employing techniques like white-box testing, unit tests help developers identify issues quickly, providing a foundation for robust and maintainable software. Furthermore, unit tests serve as a form of documentation, illustrating how each unit should function and contributing to overall code quality and reliability.
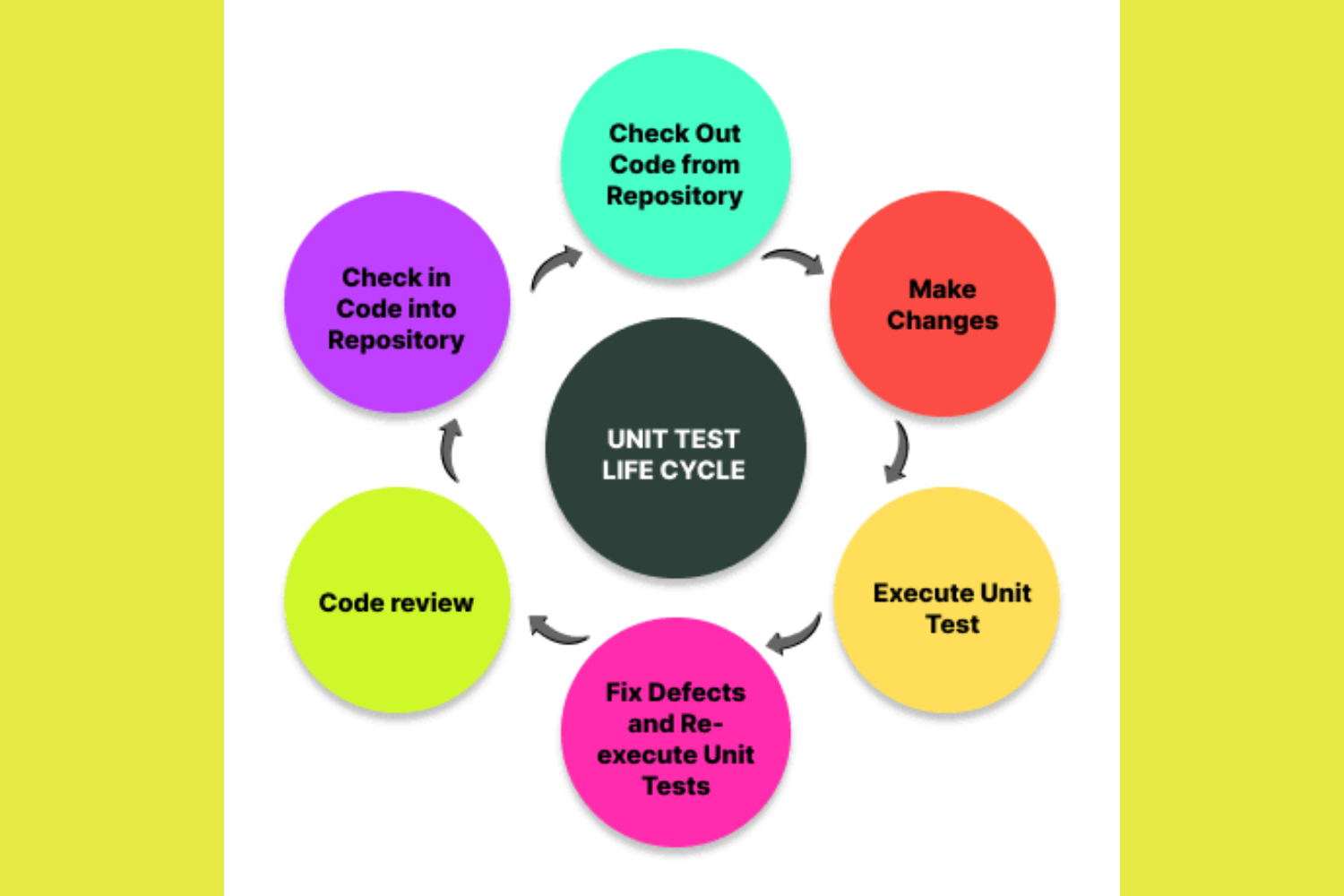
How Unit Tests Work?
Here’s a simplified overview of how unit tests work:
1. Select a Small Unit: Choose a small, self-contained part of your code to test, such as a function or method.
2. Write Test Cases: Create specific scenarios or test cases that cover different aspects of the unit’s behavior. These test cases should include input values and expected output.
3. Set Up Testing Environment: If needed, set up a testing environment or create mock objects to isolate the unit being tested from external dependencies.
4. Run Tests: Execute the test cases using a testing framework or a simple testing script.
5. Assertions: In each test case, use assertions to check if the actual output matches the expected output.
6. Pass or Fail: If all test cases pass, the unit is working as expected. If any test case fails, it means there’s an issue in the unit’s functionality.
7. Repeat and Refactor: If a test fails, investigate the problem, fix the code, and re-run the test. Iterate this process until all test cases pass.
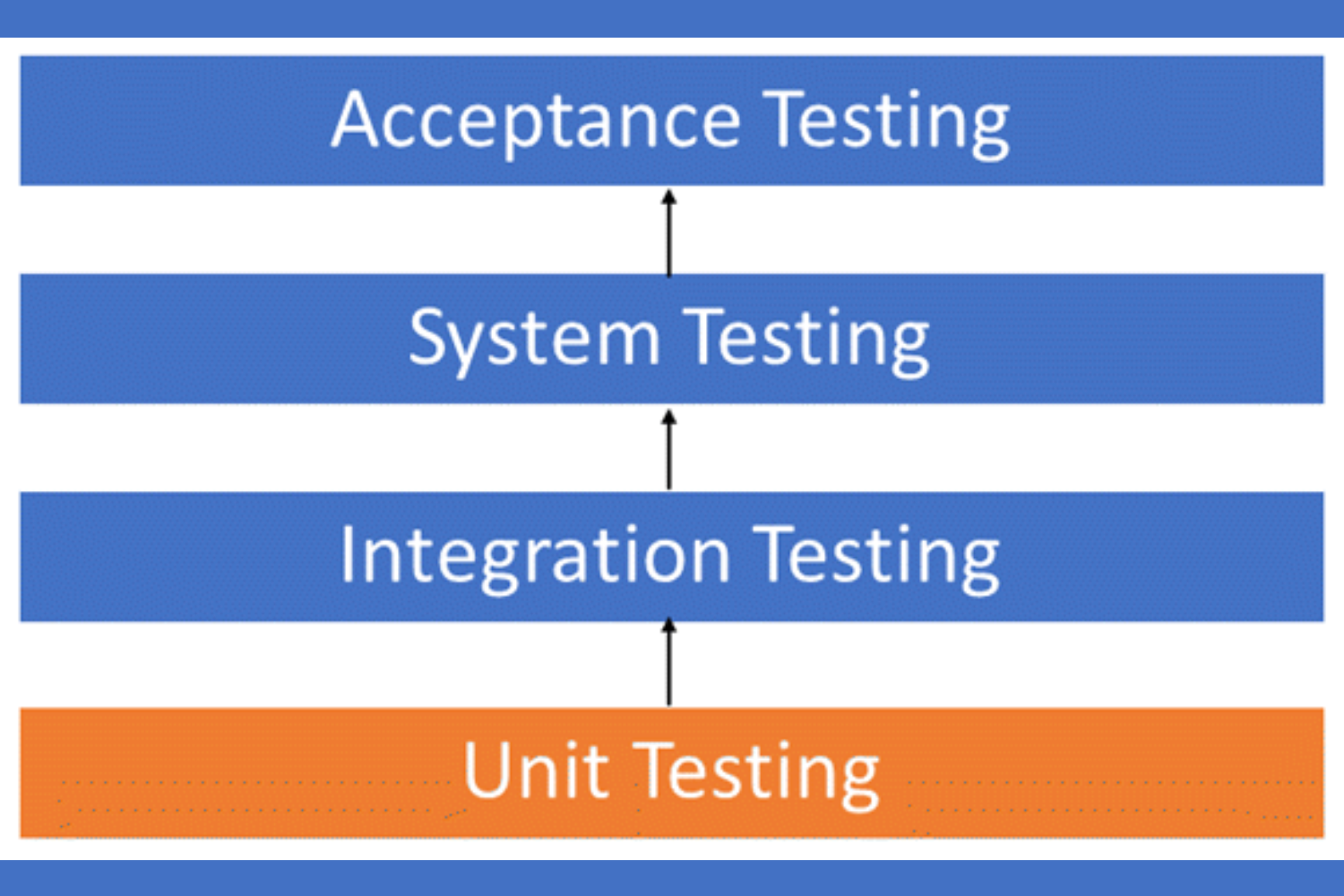
8. Automate and Maintain: Automate the testing process to run tests automatically, especially when you make changes to the code. Keep your unit tests up to date as your code evolves
Principles Of Unit Testing:
Here are 8 principles of unit testing:
1. Isolation: Unit tests are designed to isolate the specific component being tested. This means that external dependencies, such as databases, network services, or other components, are often replaced with mock objects or stubs to control the environment in which the unit test runs.
2. Automated: Unit tests are automated, meaning they can be run automatically by testing frameworks without human intervention. This automation ensures that tests can be executed frequently and consistently.
3. Repeatable: Unit tests should produce consistent and repeatable results. Running the same test multiple times should yield the same outcome if the unit being tested has not changed.
4. Fast: Unit tests are typically fast to execute. This allows developers to run them frequently during development, making it easier to catch and fix issues early in the development process.
5. Independent: Unit tests should not rely on the state or behavior of other units or external systems. Each unit test should be self-contained.
6. White Box Testing: Unit testing often involves examining the internal structure of the code being tested. Testers have access to th and can design tests that exercise various code paths, including edge cases and error conditions
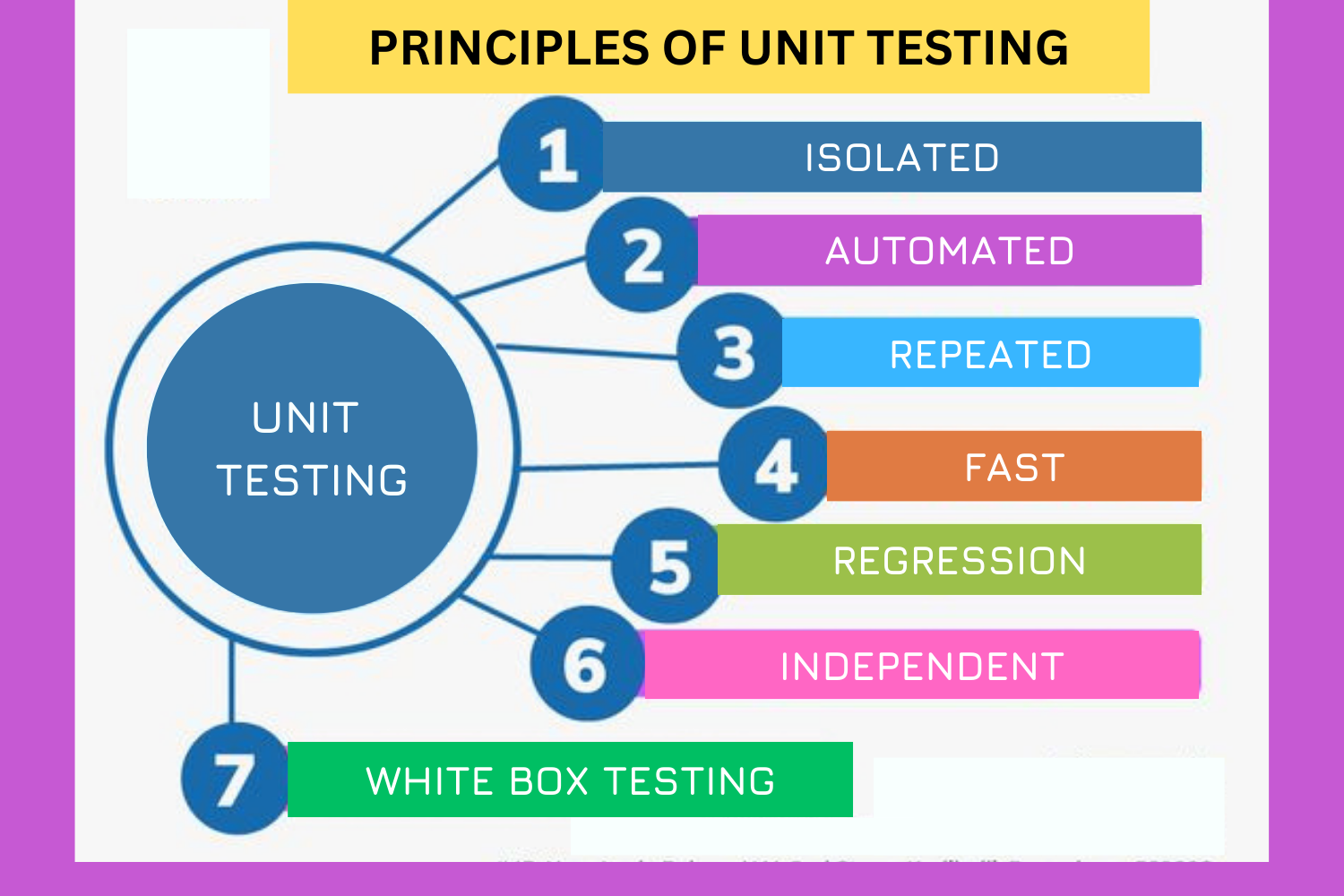
7. Regression Testing: Unit tests serve as a form of regression testing, ensuring that changes or updates to the codebase do not introduce new bugs or break existing functionality.
8. Documentation: Unit tests can serve as documentation for how a particular unit of code is expected to work, making it easier for other developers to understand and maintain the code.
Unit testing is a fundamental practice in many software development methodologies, such as Test-Driven Development (TDD) and Extreme Programming (XP). It helps improve code quality, reduces the likelihood of bugs, and makes it easier to maintain and refactor software over time. Popular unit testing frameworks and libraries, such as JUnit (for Java), NUnit (for .NET), and pytest (for Python), are commonly used to create and run unit tests.
Why perform Unit Testing?
Unit testing holds a crucial significance in the software development process. Some developers may attempt to cut corners by minimizing unit testing, but this approach is a misconception. Inadequate unit testing can result in increased costs associated with defect resolution during system testing, integration testing, and even beta testing after the application has been constructed. Conversely, when thorough unit testing is carried out during the early stages of development, it ultimately saves both time and money in the long run.
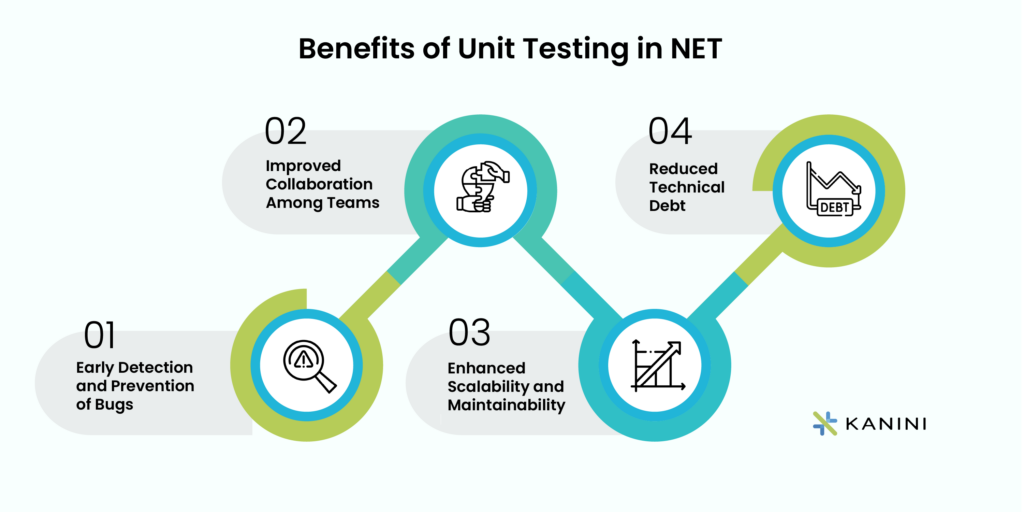
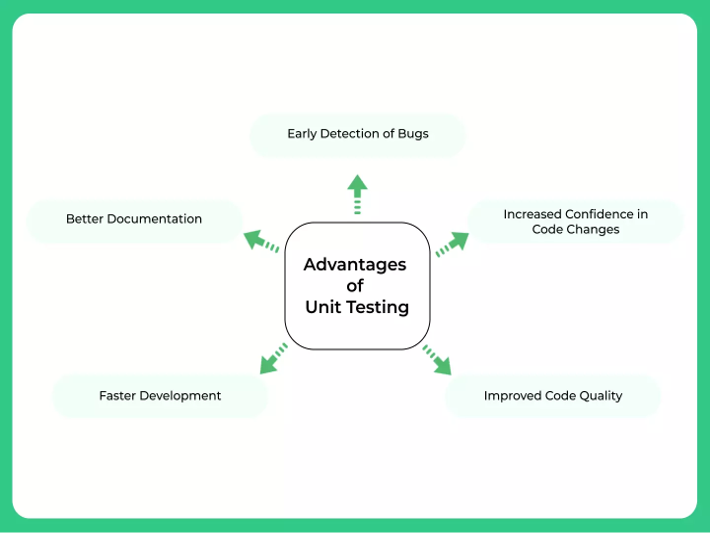
Unit testing simplifies software development by providing the following advantages:
1. Early Bug Detection: It helps catch and fix bugs early in the development process.
2. Improved Code Quality: Encourages clean and maintainable code.
3. Regression Testing: Identifies issues when code changes to prevent regressions.
4. Documentation: Serves as documentation for how code should behave.
5. Isolates Issues: Makes it easier to pinpoint the source of problems.
6. Facilitates Refactoring: Provides confidence when making code changes.
7. Supports Continuous Integration: Integrates testing into the development process.
8. Enhances Collaboration: Enables multiple developers to work together.
9. Reduces Debugging Time: Speeds up the debugging process.
10. Cost Savings: Saves time and money in the long run.
Unit Testing Tools:
Unit testing is an essential part of the software development process, and there are various tools available for different programming languages and frameworks to help developers write and execute unit tests. Here are some popular unit testing tools:
The following are some of the tools,
JUnit, Jasmine, TestNG, PHPUnit, Mocha, TestNG, AVA, Ginkgo, Cypress
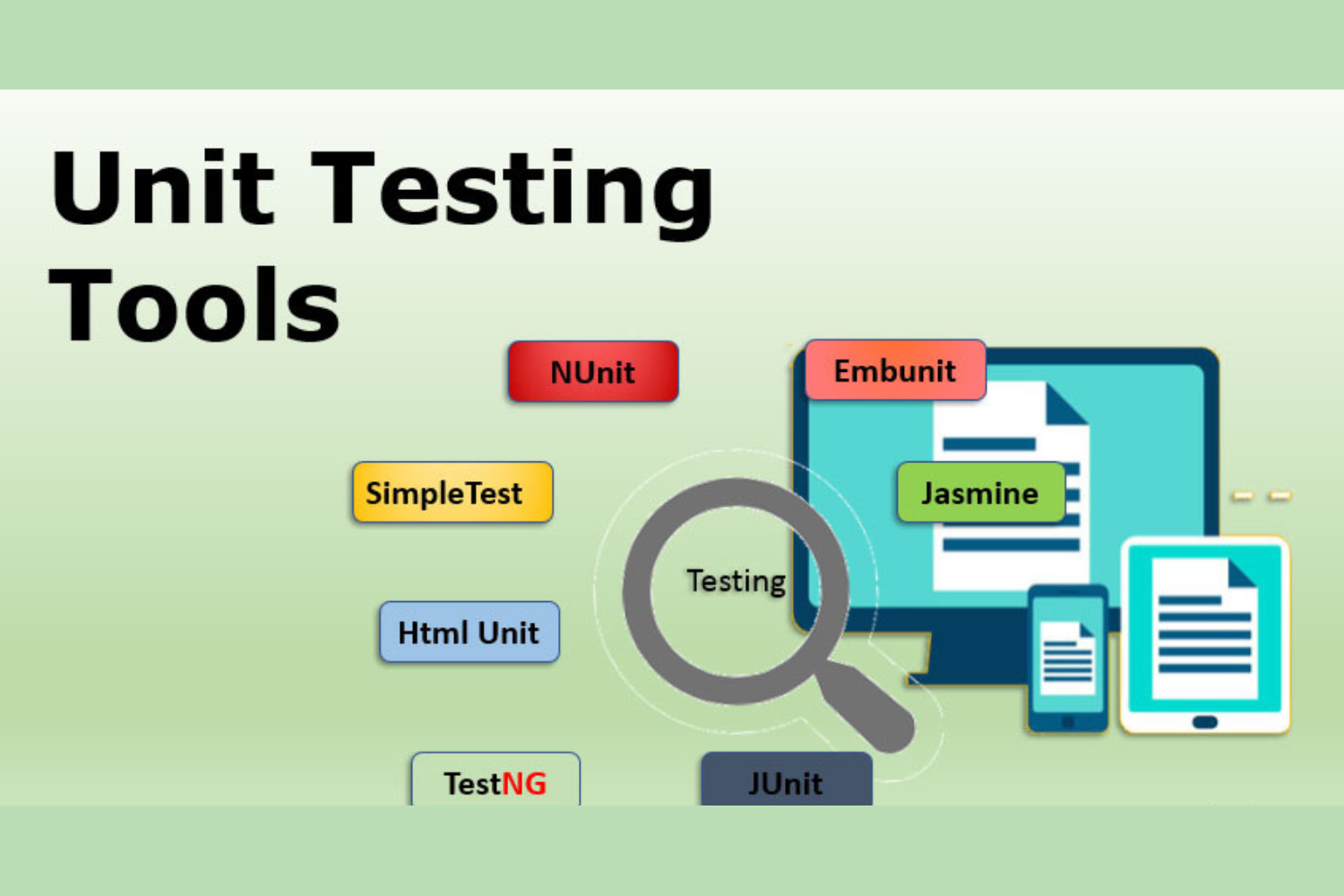
Unit Testing Types:
Unit testing can be categorized into different types based on the aspects of the code that are being tested and the goals of the tests. Some common types of unit testing include:
1. White Box Testing: In white box testing, the tests are designed with knowledge of the internal structure and implementation of the code being tested. Test cases are created to exercise specific code paths, branches, and conditions to ensure thorough coverage. It aims to test the logic and code structure.
2. Black Box Testing: Black box testing, on the other hand, treats the code as a “black box” with no knowledge of its internal workings. Test cases are designed based on the expected input-output behavior of the code. This type of testing focuses on testing the functionality and correctness of the code without looking at its implementation details.
3. Positive Testing: Positive testing, also known as “happy path” testing, involves testing the code with valid input data. It checks if the code behaves as expected when everything is correct. It is used to verify that the code meets its intended functionality under normal conditions.
4. Negative Testing: Negative testing involves testing the code with invalid or unexpected input data. It checks how the code handles errors, edge cases, and exceptional conditions. Negative testing is essential for identifying and addressing error-handling and robustness issues.
5. Boundary testing focuses on testing the code at the edge conditions or limits of its input data. It helps uncover issues related to boundary conditions, such as off-by-one errors or incorrect handling of minimum and maximum values.
6. Mock Testing: Mock testing is used to isolate the unit under test by creating mock objects or stubs for its dependencies.
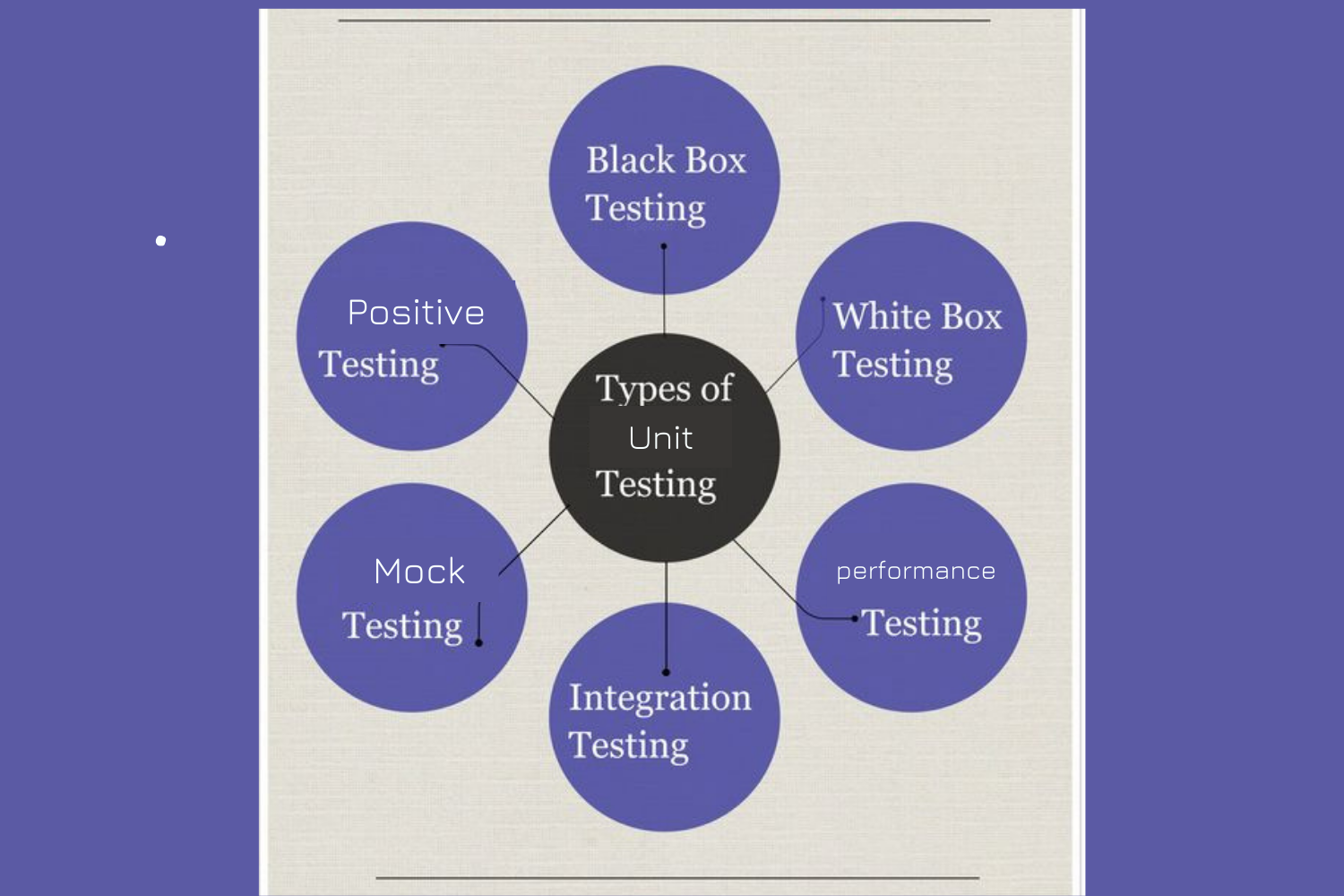
This allows for testing the unit in isolation, even when external components or services are not available or are too complex to include in the test.
7. Integration Testing: While unit testing focuses on individual units of code, integration testing verifies the interactions and compatibility of multiple units or components within a larger system. It ensures that different parts of the code work together correctly.
8. Performance Testing: Performance testing involves evaluating the unit’s response time, throughput, and resource usage under different load and stress conditions. It ensures that the unit meets performance requirements.
9. Concurrency Testing: Tests how the code behaves in multi-threaded or multi-process environments.
10. Component Testing: Focuses on testing individual components or modules.
11. State-Based Testing: Tests how the code behaves as it transitions between different states.
Unit Testing Techniques
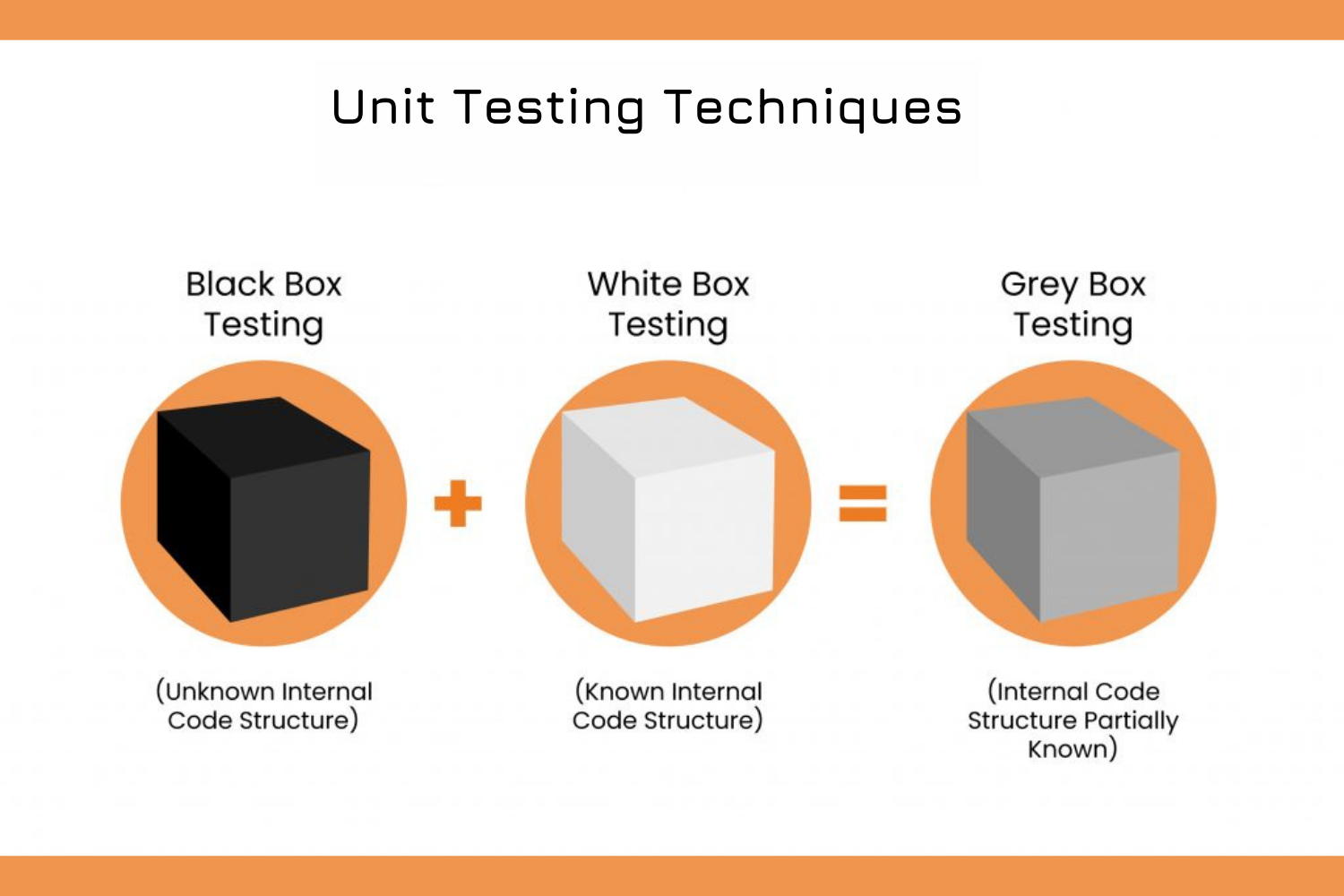
Unit testing techniques can be broadly divided into three categories:
1. Black box testing, which tests the user interface, input, and output
2. White box testing, which tests the software application’s functional behavior
3. Gray box testing, which runs test suites, test methods, test cases, and performs risk analysis.
The following is a list of code coverage strategies used in unit testing:
1. Statement Explanation
2. Coverage of Decisions
3. Coverage of Branches
4. Coverage of Conditions
5. Coverage of Finite State Machines
How is Black Box Testing performed?
Any Black Box Testing should follow the following basic methodology:
1. Having a thorough understanding of the application’s specifications using a Software Requirements Specification (SRS) document.
2. In order to save time and obtain adequate test coverage, the software is evaluated using a set of valid inputs.
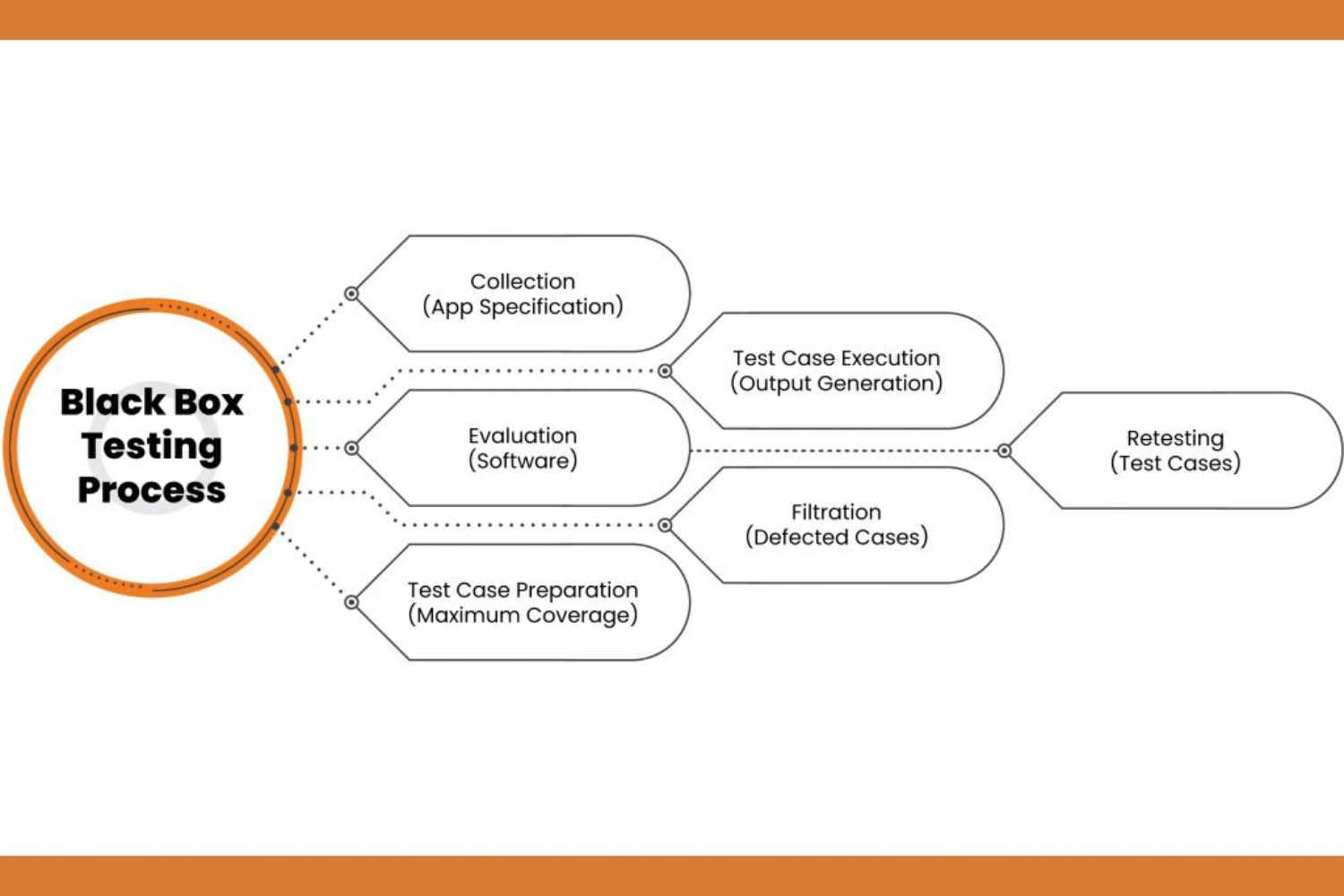
3. Preparing test cases to ensure maximum input coverage.
4. Executing test cases through the system to produce results.
5. Identifying the “Failed” steps and forwarding them to the development team for correction.
6. The test cases being retested.
How is White Box Testing performed?
1. Understanding of the Source Code
Every testing procedure starts with learning and comprehending an application’s source code. Internal testing, or “white box” testing, necessitates that testers possess in-depth knowledge of the programming languages used in the applications they are testing. Given that security is the primary reason for testing, it is imperative that the tester understands secure coding practices.
To prevent attackers from taking advantage of the vulnerabilities and inserting malicious code into the application, the tester must be able to recognize and locate security issues within the application’s code.
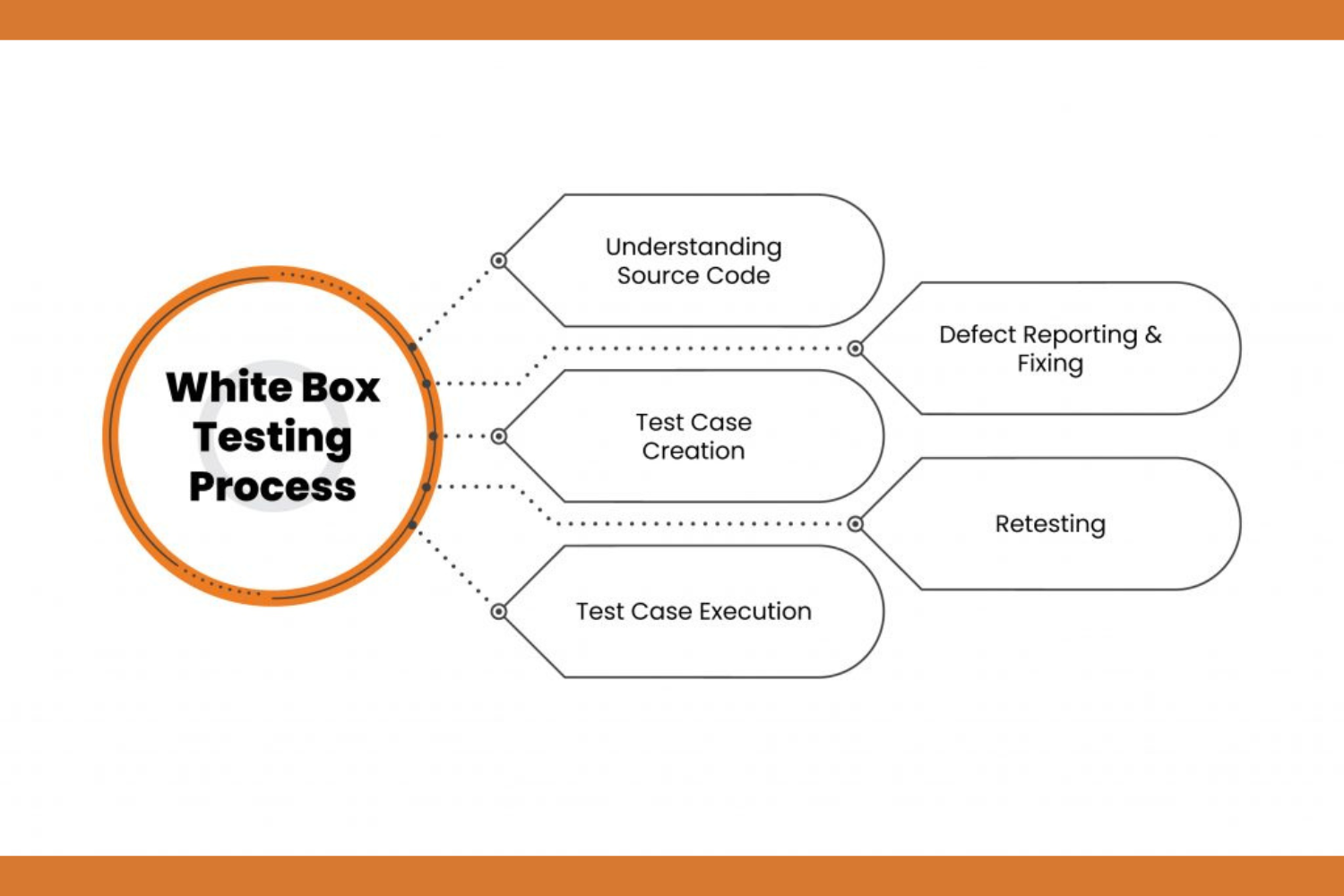
2. Test Case Creation and Execution
Testing the application’s code to ensure that it follows a proper flow and structure is the next fundamental step. Writing extra code to enable testing of the application’s code is one method to accomplish this. The developer uses this method, which necessitates a profound technical understanding of the code. The alternative approach uses trial and error, manual testing, and a variety of tools, including MobSF, BurpSuite, Dex2Jar, and many more, to carry out the testing process.
How is Grey Box Testing Performed?
1. Identifying and choosing the inputs for Black and White box testing techniques, as well as confirming the expected results from these inputs.
2. Determining the main avenues for the testing phase and comprehending the deep-level testing sub-functions.
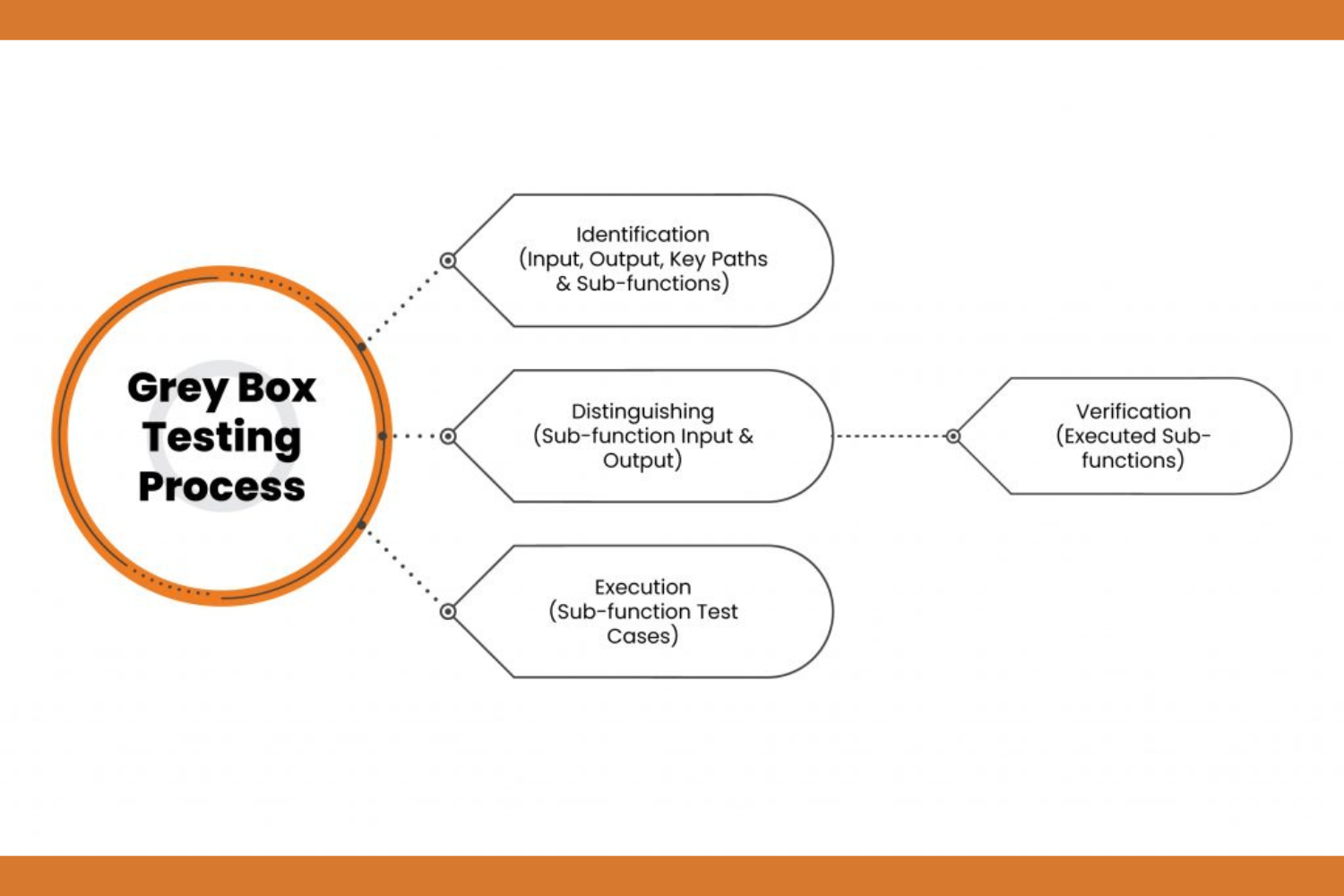
3. Determining the probable sources and destinations of the sub-functions’ inputs and outputs.
4. Carrying out sub-function test cases and confirming the results.
Career Path And Opportunities in Unit Testing:
Here are some job opportunities related to unit testing
1. Software Developer/Engineer
2. Test Engineer/QA Engineer
3. Automation Tester
4. DevOps Engineer
5. Software Test Lead/Manager
6. Quality Assurance Analyst
7. SDET (Software Development Engineer in Test)
8. Test Consultant
9. Freelance/Contractor
10. Academic/Researcher
Salary Package of Unit Testing
Unit Testing Engineer salary in India ranges between ₹ 13LPA-35LPA.
Course Highlights/ Details
1. Suited for students, fresher’s, professionals, and corporate employees
2. Live online classes
3. 4-month program
4. Certificate of completion
5. Decision Oriented Program of Analysis
6. Live Classes by highly experienced faculties
7. Hands-on experience with real-life case studies.
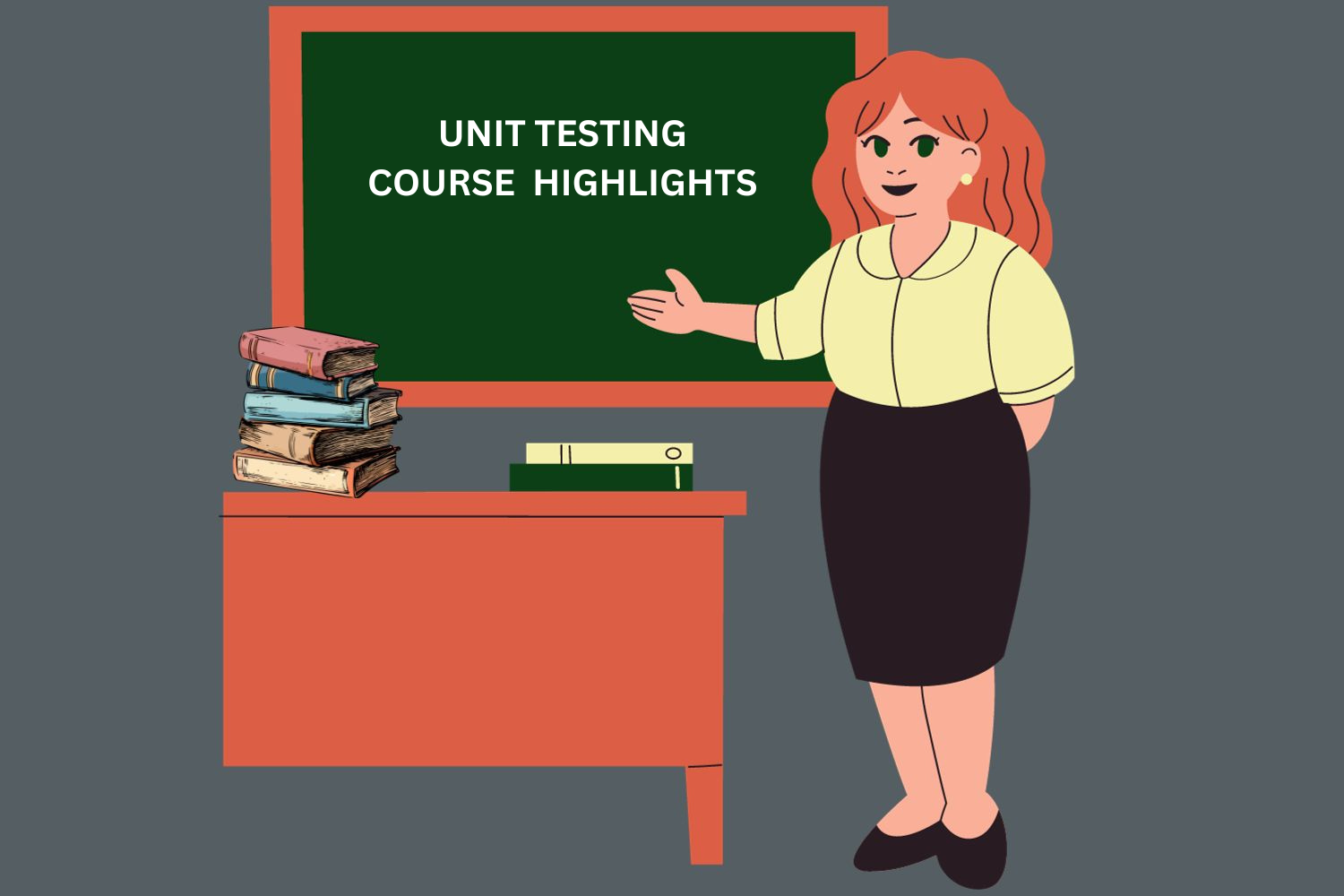
Conclusion
In conclusion, unit testing is a fundamental and indispensable practice in software development. It involves the systematic testing of individual code components in isolation to ensure their correctness and reliability. Unit tests not only serve as a safety net for identifying and rectifying bugs early in the development process but also contribute to the overall quality and stability of the software. By isolating specific units of code and subjecting them to various test scenarios, unit testing helps maintain code integrity, provides valuable documentation, and supports the efficient development process. In conjunction with other testing methodologies and practices, unit testing forms a crucial part of a comprehensive quality assurance strategy, ultimately leading to the creation of more robust and maintainable software applications.